Java pROGRAMmING
INTRODUCTION
Credge brings you the evergreen Java certification course. This is specially designed for high-school students to help them step into the world of programming. Learn right from the fundamentals to the complex Java concepts like Hashing, Stacking, Arrays, Huffman coding, etc., and become an on-demand programmer.
Course Duration
6-8 weeks
Mode
Live Interactive Classes
Who is it for?
High School Students
Salient features
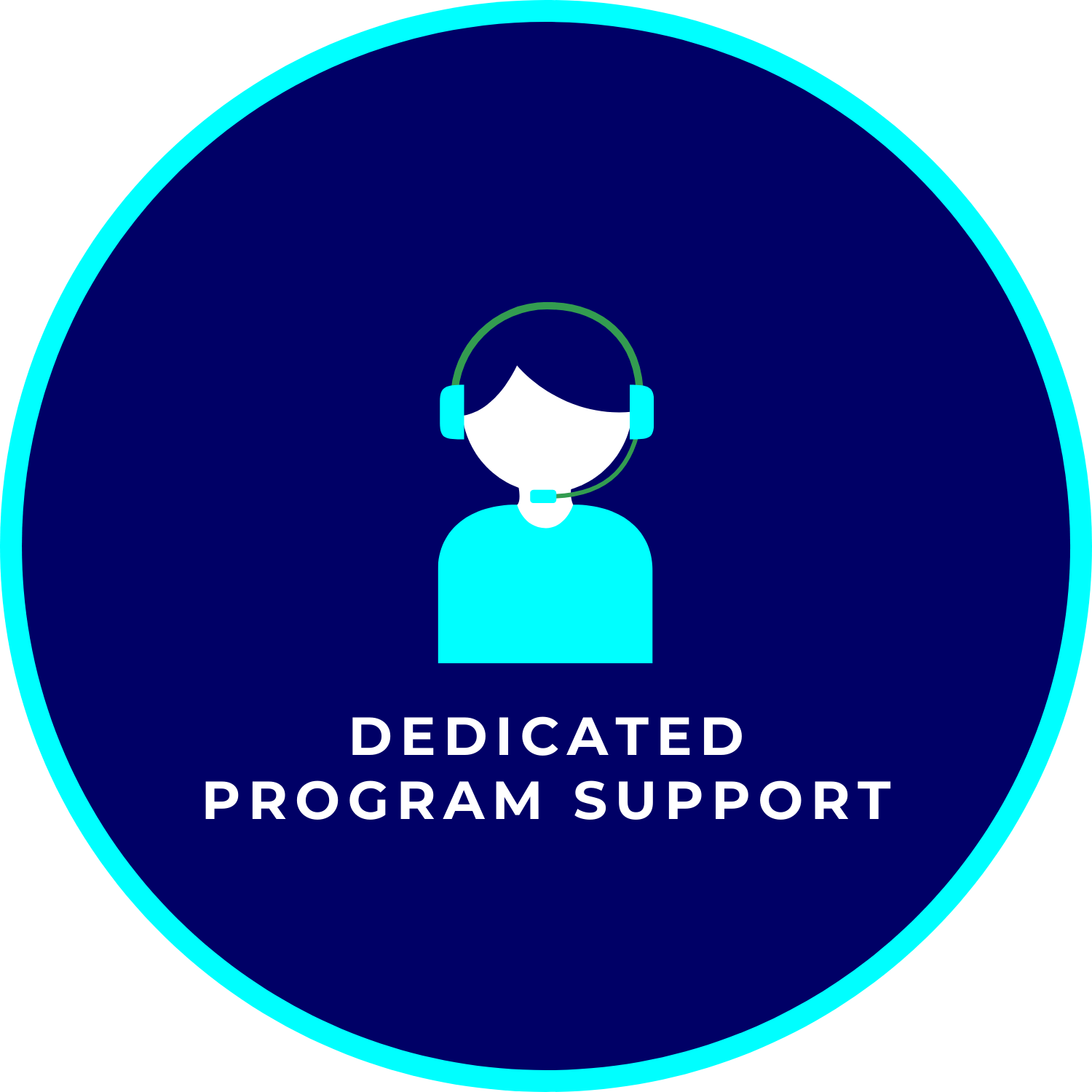
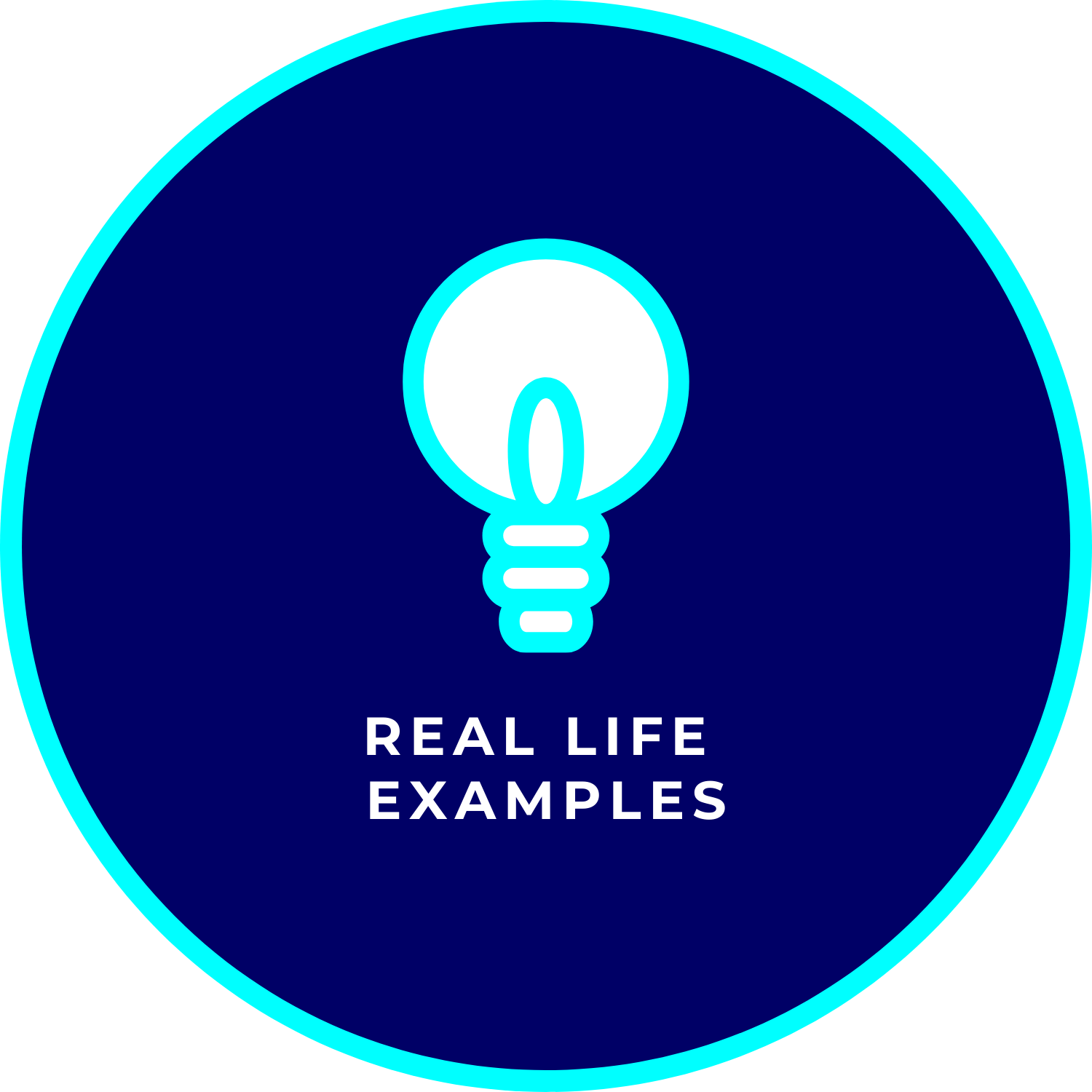
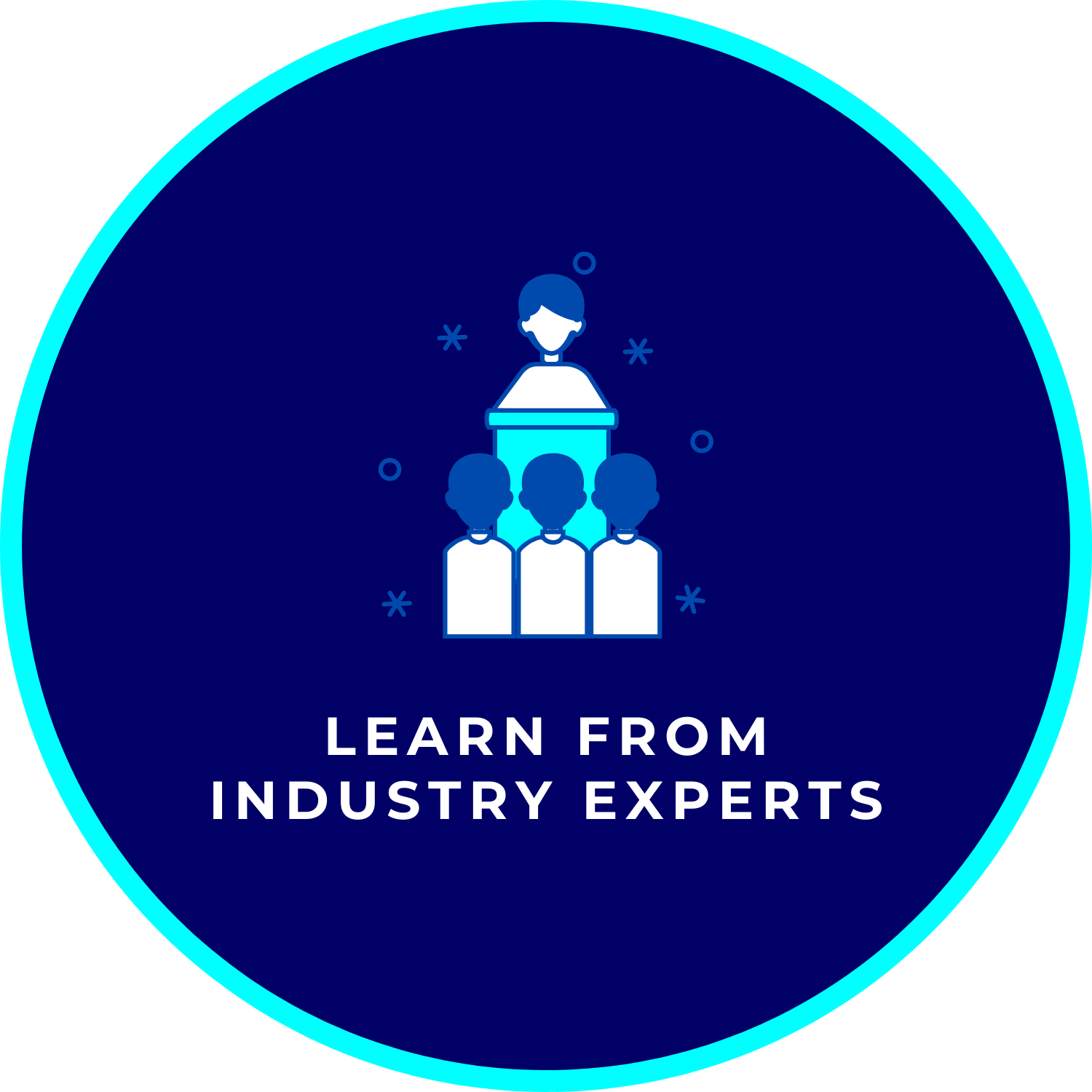
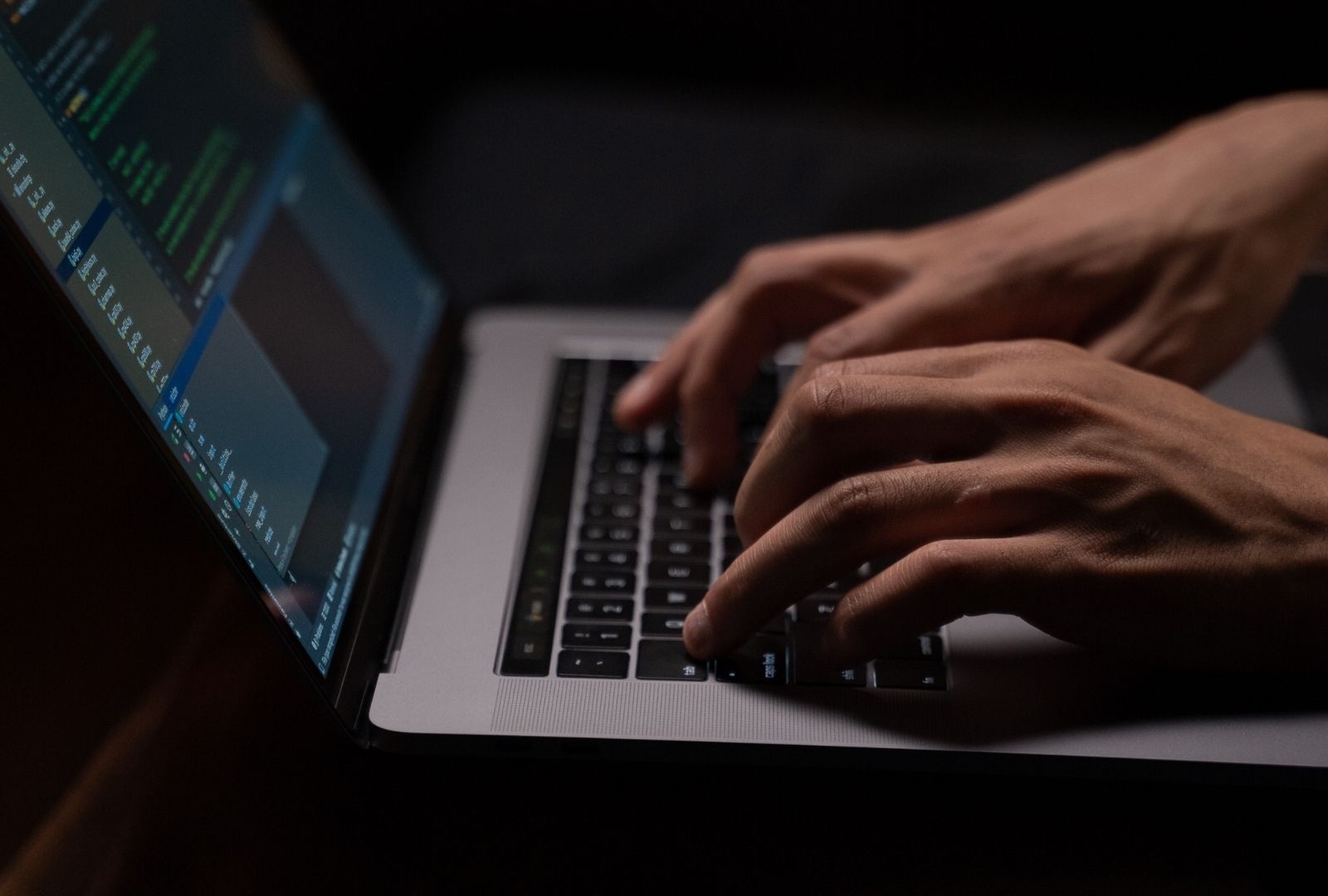
Why Choose this Program?
Advantage in College Admissions
Add Honors Program to your Resume
Complete College Pre-requisites
Explore Areas of Interest
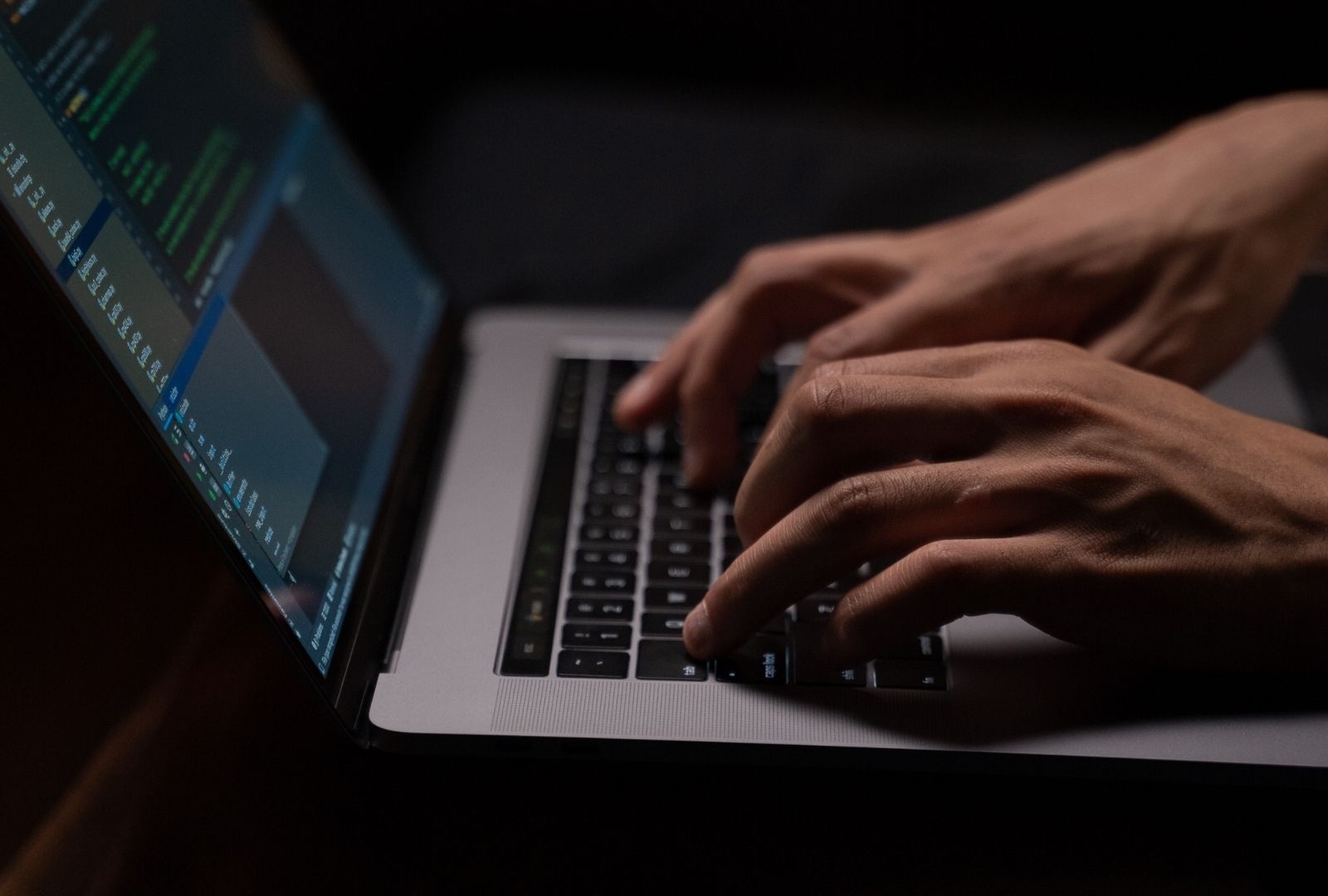
Why Choose this Program?
Advantage in College Admissions
Add Honors Program to your Resume
Complete College Pre-requisites
Explore Areas of Interest
What do Experts have to say
Have the master minds convince you. Know the future of the course you sign up for and Get set to grow. Apply Now!
Curriculum
Module 1: Basic Java
-
- Flowcharts
- Concepts in Java
- Java Platform Independence
- Installation of Java
- Hello World and Variables
- Taking Input
- Storing Data
- Explicit Typecasting
- Operators
- Loops and Conditionals
- Conditionals
- While Loop
- For Loop
- Break and Continue
- Patterns
- Square Pattern
- Triangular and Character Patterns
- Reverse Number Patterns
- Mirror Number Patterns
- Star Patterns
- Diamond Star Pattern
- Scope of Variables
- Operators
- Bitwise Operators
- Power set using Bitwise
- Post and Pre Increment Operator
- Post and Pre Decrement Operator
- Mathematics
- Factorial of Number
- Trailing zeros in Factorial
- GCD or HFC, LCM of two numbers
- Prime Factors
- All Divisors of a Number
- Iterative Power
- Computing Power
- Functions
- What are Functions?
- How does Function Calling work?
- Pass By Value
Module 2: OOPS
- Intro to OOPS
- Constructors and Modifiers
- Components of OOPS
- Inheritance
- Private Members
- Protected and Super Keywords
- Inheritance and Constructors
- Polymorphism
- Exception Handling
- Final Keyword
- Method Overriding
Module 3: Arrays
- Intro to Arrays
- How Arrays are Stored?
- Operations on Arrays
- Passing Arrays to Function
Module 4: Searching, Sorting and Multidimensional Arrays
- Intro to Binary Search
- Selection Sort
- Bubble Sort
- Insertion Sort
- Quick Sort
- Merging Sorted Arrays
- Strings and how to store them
- 2D Arrays and it’s Sorting
- Passing 2D as Arguments
- Jagged Arrays
Module 5: Everything about Time And Space Complexity
- Asymptotic Notation
- Omega Notation
- Theta Notation
- Analysis of Common loops
- Linear Search Time Complexity
- Insertion Sort Complexity
- Merge Sort Complexity
- Selection Time Complexity
- Fibonacci Space Complexity
Module 6: Recursion
- Method Overloading
- Variable Arguments
- What is Recursion?
- Application of Recursion
- Analysis of Recursion Time Complexity
- Recursion and PMI Method
- Recursion and Arrays
- Helper Functions
- Practise Questions
Module 7: OOPS 2.O
- Dynamic Method Dispatch
- Abstract Function and Classes
- Interfaces
- Generics
- About Generics
Module 8: Hashing
- Intro to Hashing
- Hashing Application
- Direct Address table
- Chaining VS Open Addressing
- Inbuilt Hashmap
- Bucket Array and Hash Function
- Collision handling
- Time Complexity and Load Factor
- Rehashing
- Practise Questions
Module 9: Linked List
- Array List
- Intro to Linked List
- Print Linked list
- Taking linked list input
- Inserting node in Linked list
- Deleting Node in Linked list
- Midpoint of Linked List
- Merge Sort
- Merging two sorted LL
- Reverse LL
- Reverse Recursively Double Node
- Reverse Iterative
- Insert Recursively
- Delete Recursively
- Variations of LL
- Circular doubly LL
- Traversal in LL
- Linked List in Collections
Module 10: Stacks
- Intro to Stacks
- Stacks Using Array
- Stack double capacity
- Stack using LL
- Stack in Collections
- Balanced Parenthesis
- Intro to Queue
- Queue using Array
- Dynamic Queue
- Queue using LL
- Queue in Collections
- Dequeue
- Array Implementation of Dequeue
- Array Dequeue
Module 11: Trees
- Tree Data Structure
- Applications of Tree
- Tree node class
- Finding the number of nodes
- Node with the largest data
- Height of Tree
- Depth of node
- Maximum in Binary Tree
- Tree Traversals
- Binary Tree
Module 12: Binary Tree
- What is a Binary Tree?
- Count nodes in Binary Tree
- Take Tree input and print recursively
- Find Node
- Height of Tree
- Diameter Binary Tree
- Preorder and Postorder Traversal
- Maximum in Binary Tree
- LCA of Binary Tree
- Serialise and Deserialize a Binary Tree
- Iterative Inorder Traversal of Binary tree
Module 13: Priority Queues
- What is the Priority Queue?
- Ways to implement Priority Queues
- Heaps Introduction
- CBT Height and its implementation
- Insert and Delete in Heaps
- Implementation – Insert
- Remove Min Solution and Complexity Analysis
- Inplace Heap Sort
- Inplace Heap Sort – Solution
- Inbuilt Priority Queue and K sorted array
- K largest elements
- Inbuilt Max Priority Queue
Module 14: Tries and Huffman Coding
- Intro to Tries
- Tries Implementation – Insert a Word
- Tries Implementation – Searching and Deleting a Word
- HashMap vs Tries
- Types of Tries
- Huffman Coding
Module 15: Dynamic Programming
- Minimum coins to make a value
- Minimum Cost path- Recursive, Memoization, DP
- LCS – Memoization, DP
- Optimal Strategy for a Game
- Maximum sum with no consecutive
- Matrix Chain multiplication
- Allocate minimum pages
- Edit Distance-Recursive, Memoization, DP
- Knapsack
Module 16: Graph
- Graph Introduction
- Graph Representation
- Adjacency List Implementation
- Adjacency Matrix
- BFS Traversal
- Applications of BFS
- DFS Traversal
- Applications of DFS
- Topological Sorting (DFS Based Algorithm)
- Implementation of Dijkstra_s Algorithm
- Kosaraju’s Algorithm
- Bellman Ford Shortest Path Algorithm
- Articulation Point
- Kruskal’s AlgorithmBellman-Ford
Module 17: Greedy
- Selection Activity Problem
- Fractional Knapsack
- Job Sequencing Problem
Module 18: Backtracking
- Rat in Maze
- Sudoku Problem
Projects
Our Core Java Training course aims to deliver quality training that covers solid fundamental knowledge of core concepts with a practical approach. Such exposure to the current industry use-cases and scenarios will help learners scale up their skills and perform real-time projects with the best practices.
You work on building websites, software applications.
- Build a New Bank Account Application
- Build a Student Database Application
- Build an Email Administration Application
- Online Reservation System
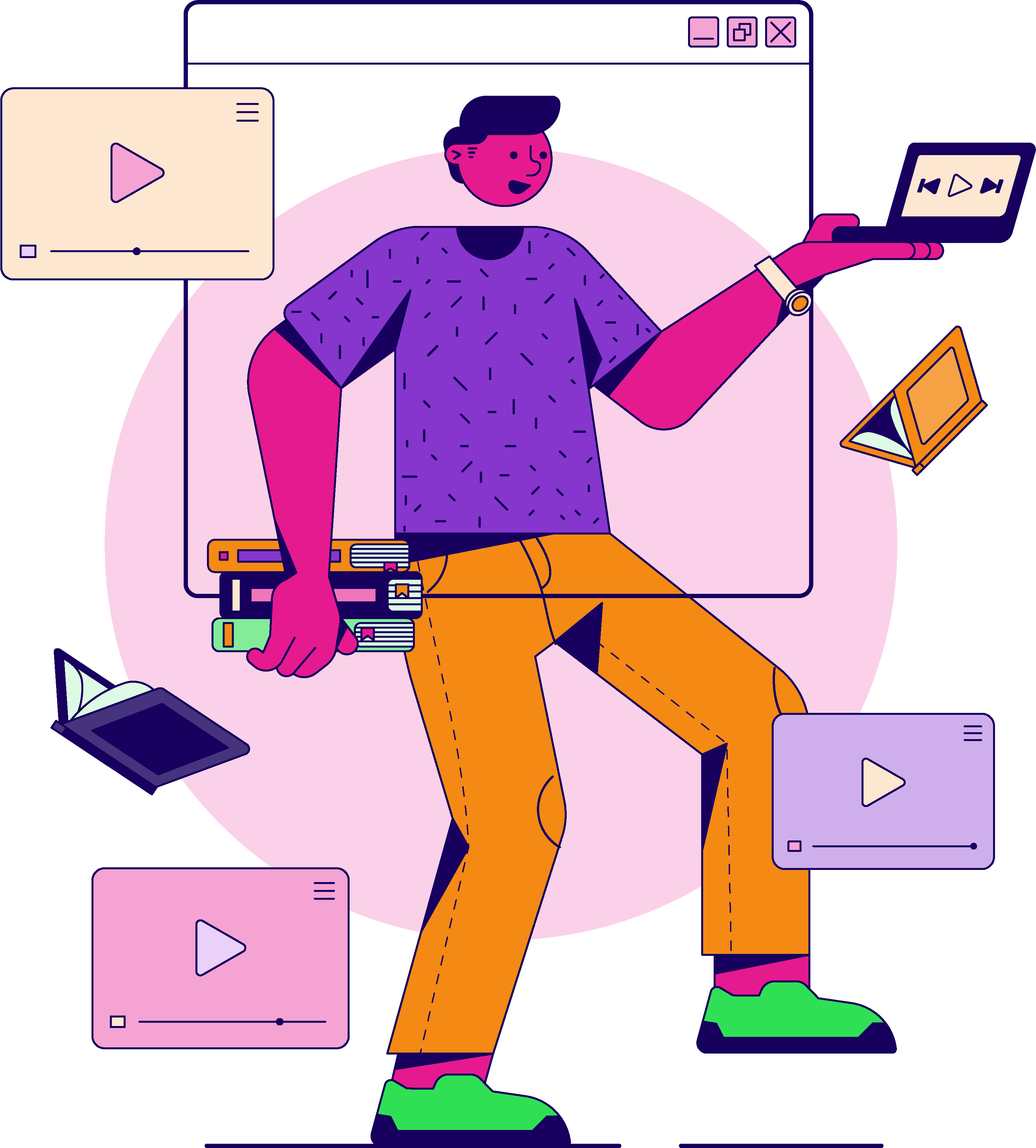
Our stats
TOP 50 US AND TOP 20 UK ACCEPTANCES
IVY LEAGUE ACCEPTANCES
UNIVERSITY OF CALIFORNIA OFFERS
PROFESSIONAL PROGRAMS
%
PROFESSIONAL PROGRAMS
You’re Next Big Question
WHY US?
Learner-centered education for all!
We aim to bring in a tailored curriculum that provides learner-centered education to all our mentees. We’re on a mission to make our learners globally proficient by helping them harness their ultimate potential.
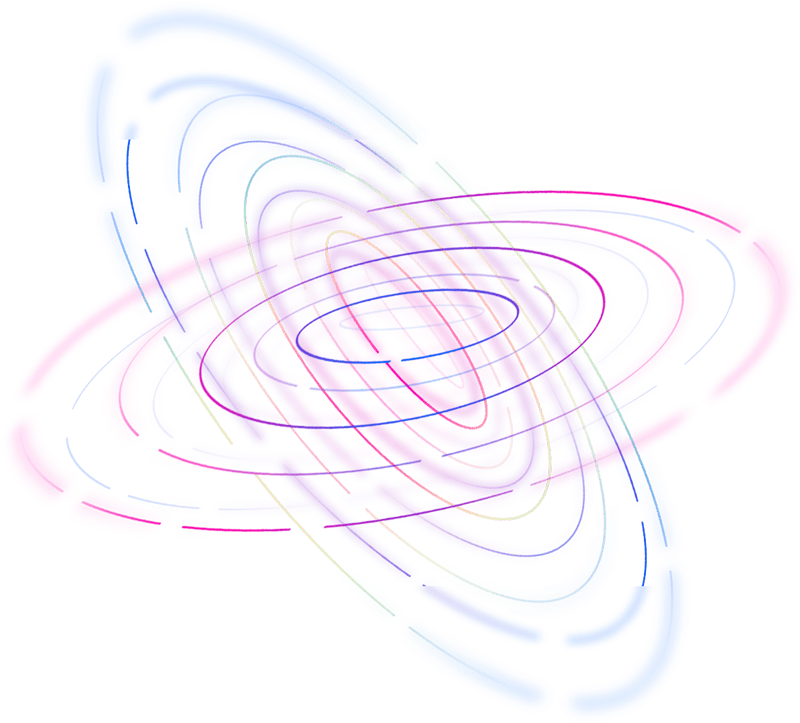

Evaluate your growth efficiently
You get to have access to top-notch evaluation, incorporated with instructions and feedback to improvise yourself.

Learning with adaptive technology tailored to your needs
Power ahead your learning with the brand new adaptive technologies in the market

Get mentored by mentors from prestigious companies
Get access to hone your knowledge and skills by getting personalized guidance from mentors of some world-class companies.

Insight dashboards for parents/students and professionals
Get access to your personalized learning dashboard to keep track and analyze your skills and growth.
similar programs
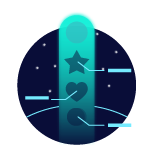
Artificial Intelligence
Understand the basics of the advanced concepts like Deep Learning, Keras, Tensorflow Convolutional NN, Vectorization and a lot more
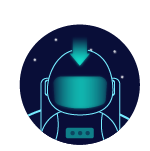
Digital Marketing
Learn the in and outs of digital marketing like SEO, Website Analytics, Content Creation, Branding, FB and Google Ads and you’re all set to don different hats.
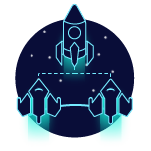
Data Science
An opportunity to learn to analyse Data, perform Statistical Modelling ,understand the different Regression Techniques and apply your Data Science knowledge